The ChatGPT API by OpenAI is a powerful tool that enables developers to integrate advanced AI capabilities into their applications, products, or services. This complete guide will walk you through the ins and outs of the ChatGPT API, including how to get started, its features, use cases, best practices, and common pitfalls to avoid. With its detailed explanations and clear organization, this guide is designed to be your go-to resource for understanding the ChatGPT API.
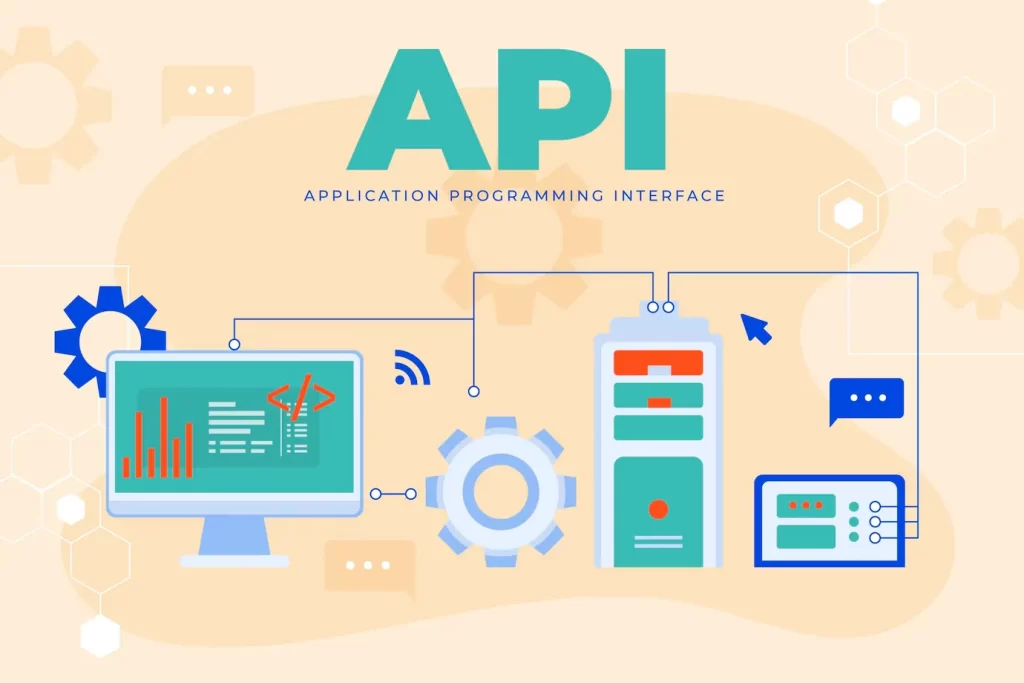
Table of Contents
- Table of Contents
- 1. Understanding ChatGPT
- 2. Setting Up the ChatGPT API
- 3. API Usage and Features
- 3.1. Sending a Request
- 3.2. Receiving and Handling Responses
- 3.3. Prompts and User Inputs
- 3.4. Modifying API Parameters
- 4. Use Cases and Examples
- 4.1. Content Generation
- 4.2. Virtual Assistants
- 4.3. Language Translation
- 4.4. Sentiment Analysis
- 4.5. Code Generation
- 4.6. Tutoring and Learning Assistance
- 5. Best Practices
- 6. Common Pitfalls and Solutions
- 7. Frequently Asked Questions
- 8. Conclusion
- 9. Additional Resources
Table of Contents
1. Understanding ChatGPT
ChatGPT, short for Chatbot Generative Pre-trained Transformer, is an advanced language model developed by OpenAI. It is based on the GPT-4 architecture and is designed to understand and generate human-like text. This powerful AI tool can be used for a wide range of tasks, from generating content to answering questions, translating languages, and much more.
The ChatGPT API allows developers to access and utilize the capabilities of ChatGPT within their applications, products, or services. It provides a simple, straightforward interface for interacting with the ChatGPT engine.
2. Setting Up the ChatGPT API
Before you can start using the ChatGPT API, you need to set it up by meeting certain prerequisites, creating an API key, and installing the necessary tools.
2.1. Prerequisites
To use the ChatGPT API, you need to have the following:
- An OpenAI account
- API access enabled on your account
- Python 3.6 or later
- Basic knowledge of Python programming and RESTful APIs
2.2. Creating an API Key
To create an API key, follow these steps:
- Log in to your OpenAI account.
- Navigate to the API settings page.
- Click on “Create new API key.”
- Provide a descriptive name for the key and select the desired permissions.
- Click “Create” to generate the key. Make sure to copy and save the key securely, as you won’t be able to access it again.
2.3. Installation
To install the required packages, open your command prompt or terminal and run the following command:
pip install openai
This will install the OpenAI Python library, which provides a convenient wrapper for interacting with the ChatGPT API.
3. API Usage and Features
The ChatGPT API offers a variety of features that allow developers to harness its natural language understanding and generation capabilities. In this section, we will dive deeper into the API usage and features, focusing on sending requests, receiving and handling responses, working with prompts and user inputs, and modifying API parameters.
Now that you have set up the ChatGPT API, it’s time to explore its features and learn how to use it effectively.
3.1. Sending a Request
To interact with the ChatGPT API, you need to send a request with a prompt or a series of user inputs. Here’s a basic example of how to send a request using Python:
import openai openai.api_key = "your_api_key # Set up the prompt and API parameters prompt = "Write a short introduction to ChatGPT." max_tokens = 100 temperature = 0.8 # Send the request to the API response = openai.Completion.create( engine="text-davinci-002", prompt=prompt, max_tokens=max_tokens, temperature=temperature, n=1, stop=None, ) # Extract and print the generated text generated_text = response.choices[0].text print(generated_text)
Replace your_api_key
with your actual API key, and run the code to generate a short introduction to ChatGPT.
3.2. Receiving and Handling Responses
When you send a request to the ChatGPT API, it returns a response that contains the generated text. The response is in JSON format, and you can access the generated text using the response.choices[0].text
attribute, as shown in the previous example.
You can also extract other information from the response, such as the token count, timestamp, or model used. For example:
token_count = response.usage["total_tokens"] timestamp = response.timestamp model = response.model
3.3. Prompts and User Inputs
You can use prompts or user inputs to guide the ChatGPT API in generating relevant text. For conversational applications, you can provide a series of messages as user inputs, with each message having a ‘role’ (either ‘system’, ‘user’, or ‘assistant’) and ‘content’ (the message text). Here’s an example of how to send user inputs:
messages = [ {"role": "system", "content": "You are an assistant that helps users with their daily tasks."}, {"role": "user", "content": "What's the weather like today?"}, ] response = openai.Completion.create( engine="text-davinci-002", messages=messages, max_tokens=50, temperature=0.8, ) generated_text = response.choices[0].text print(generated_text)
3.4. Modifying API Parameters
There are several parameters you can modify to control the behavior of the ChatGPT API, including:
engine
: The specific model to use (e.g., “text-davinci-002”, “text-curie-002”, etc.).max_tokens
: The maximum number of tokens (words or word pieces) in the generated text.temperature
: Controls the randomness of the output (higher values make the output more random, lower values make it more deterministic).n
: The number of independent completions to generate (useful for generating multiple alternative responses).stop
: A list of strings that, if encountered, will stop the generation process.
4. Use Cases and Examples
The ChatGPT API offers a wide range of applications across various domains. By integrating the API into your projects, you can harness the power of ChatGPT to create innovative and useful solutions. Here are some use cases and examples to help you understand its potential:
4.1. Content Generation
You can use the ChatGPT API to generate articles, blog posts, social media updates, or other types of content. Just provide a prompt that describes the desired content and adjust the API parameters to control the output’s length and style.
4.2. Virtual Assistants
The ChatGPT API can be integrated into virtual assistant applications to provide natural language understanding and generation capabilities. Using a series of user inputs, you can guide the assistant’s responses to user queries, making the conversation more interactive and engaging. For example:
messages = [ {"role": "system", "content": "You are a virtual assistant that helps users with their daily tasks."}, {"role": "user", "content": "What are the benefits of daily exercise?"}, ]
4.3. Language Translation
By providing a prompt that specifies the desired translation, the ChatGPT API can be used for language translation tasks. For example:
Prompt: "Translate the following English text to Spanish: 'The early bird catches the worm.'"
4.4. Sentiment Analysis
The ChatGPT API can be employed to analyze the sentiment of a given text. By providing a prompt that asks the model to determine the sentiment, it can return a response indicating whether the text is positive, negative, or neutral. For example:
Prompt: "Determine the sentiment of the following sentence: 'I absolutely loved the movie and its breathtaking visuals.'"
4.5. Code Generation
ChatGPT can also generate code snippets when given a specific programming task or problem. Provide a prompt that clearly outlines the coding task, and the API will return a relevant code snippet. For instance:
Prompt: "Write a Python function that takes a list of numbers and returns their sum."
4.6. Tutoring and Learning Assistance
The ChatGPT API can be used to create tutoring or learning assistance applications that help users understand complex topics or solve problems. Provide a prompt that asks the model to explain a concept or solve a problem, and it will return a detailed, step-by-step response. For example:
Prompt: "Explain the process of photosynthesis in simple terms."
These are just a few examples of the numerous use cases for the ChatGPT API. Its flexibility and powerful natural language understanding and generation capabilities enable developers to create a wide range of innovative applications across various domains.
5. Best Practices
To get the most out of the ChatGPT API, follow these best practices:
- Be explicit with your prompts and user inputs to guide the model effectively.
- Experiment with different API parameters to find the best combination for your specific use case.
- Use the
stop
parameter to control when the generation process should end. - Monitor the token usage in your API calls to avoid unexpected billing surprises.
- Handle errors and exceptions gracefully in your application.
6. Common Pitfalls and Solutions
Some common pitfalls when using the ChatGPT API include:
- Receiving irrelevant or nonsensical responses: Refine your prompt or user inputs to be more explicit, or adjust the API parameters (e.g., lower the temperature) to guide the model better.
- Hitting rate limits or token limits: Monitor your token usage and make sure you stay within the limits specified in your API plan.
- Inconsistency between generated outputs: Use the
n
parameter to generate multiple alternative responses and choose the most appropriate one.
7. Frequently Asked Questions
- What are the rate limits for the ChatGPT API?
Rate limits depend on your API plan. Please refer to the OpenAI documentation for the specific rate limits associated with your account. - Can I use the ChatGPT API for free?
The ChatGPT API is a paid service. You can review the pricing details on the OpenAI website. - How can I improve the quality of the generated text?
Experiment with different prompts, user inputs, and API parameters to find the best combination for your use case. Being more explicit in your instructions can also help improve the quality of the output.
8. Conclusion
The ChatGPT API is a powerful tool that allows developers to harness the capabilities of ChatGPT for various applications. By following this complete guide, you can effectively set up, use, and optimize the API for your specific needs. With its flexibility and powerful natural language understanding and generation features, the ChatGPT API can be a game-changer for your application, product, or service.
9. Additional Resources
To further deepen your understanding of the ChatGPT API and its capabilities, you can explore the following resources:
- Official OpenAI Documentation: Visit the OpenAI documentation for comprehensive information on the ChatGPT API, including detailed explanations of API parameters, rate limits, and best practices. (https://beta.openai.com/docs/)
- OpenAI Community: Join the OpenAI community to engage with other developers, share ideas, ask questions, and find solutions to common challenges. (https://community.openai.com/)
- OpenAI GitHub Repositories: Explore the OpenAI GitHub repositories for example projects, code snippets, and other resources that can help you get the most out of the ChatGPT API. (https://github.com/openai)
- Online Tutorials and Courses: Search for online tutorials, courses, or blog posts that focus on the ChatGPT API and its use cases. These resources can help you gain a deeper understanding of the API and its potential applications.
By leveraging these additional resources and continually experimenting with the ChatGPT API, you can further refine your skills and develop more advanced, robust, and innovative applications that take full advantage of the API’s capabilities. As you continue to work with the ChatGPT API, remember to stay up-to-date with the latest developments, improvements, and best practices to ensure you are using the API effectively and efficiently. Happy coding!